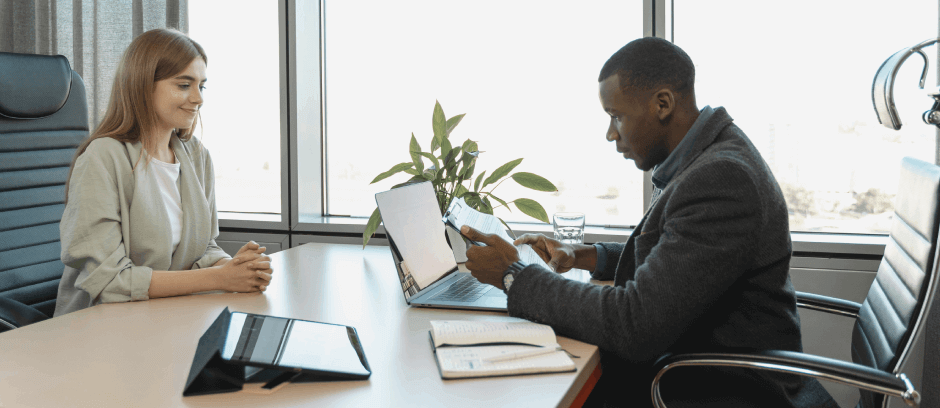
Top 60 Java Interview Questions and Answers: Ace Your Next Interview
In the realm of software development, mastering Java is akin to conquering Mount Everest. Whether you’re a budding developer or a seasoned professional seeking a career transition, Java programming questions often dominate the technical interviews. As such, we’ve compiled the top 60 Java interview questions, ranging from basic concepts of Java for interview settings to more complex queries.
Before we delve into these key questions, remember that Upskillist offers a comprehensive online Coding & Technology course. This program can serve as an excellent resource to sharpen your Java skills and enhance your professional journey.
What is Java?
Java is an object-oriented, class-based programming language developed by Sun Microsystems in 1995. It is designed to be platform-independent, following the principle of “Write Once, Run Anywhere” (WORA). This means Java code, once written and compiled, can run on any platform that supports Java without needing to be recompiled.
- What are the key features of Java?
Java has several key features:
- Object-oriented: Everything in Java is an object, which helps to model real-world problems based on objects.
- Platform independent: Unlike other languages such as C, C++, etc., which are compiled into platform-specific machines. Java is guaranteed to be write-once, run-anywhere by the Java Virtual Machine (JVM).
- Simple: Java is designed to be easy to learn and use efficiently.
- Secure: With Java’s secure feature enables the development of virus-free, tamper-free systems.
- Allocating memory: In Java, memory is divided into two parts, heap and stack, facilitating its excellent memory management.
- What do you understand by Java’s object-oriented programming paradigm?
The object-oriented programming (OOP) paradigm in Java is a methodology that simplifies software development and maintenance by providing a few concepts:
- Objects: An object is a real-world entity that has a state and behaviour.
- Class: A class can be defined as a template or blueprint that describes the behaviours/states related to the object of its type.
- Inheritance: It is a mechanism where one object acquires all the properties and behaviours of a parent object.
- Polymorphism: It is a concept by which we can perform a single action in different ways.
- Abstraction: It is a mechanism of hiding the implementation details and showing functionality to the user.
- Encapsulation: It is a mechanism of wrapping the data (variables) and code acting on the data (methods) into a single unit.
- Explain the basic concepts of Java.
Java is based on several core concepts:
- Object-Oriented Programming (OOP): Java uses objects to model real-world entities. Key OOP concepts include encapsulation, inheritance, polymorphism, and abstraction.
- Platform Independence: Through the Java Virtual Machine (JVM), Java code can run on any device and operating system.
- Syntax: Java’s syntax is similar to C and C++, making it easier for programmers from these backgrounds to learn Java.
- Automatic Memory Management: Java handles memory allocation and deallocation automatically, reducing the likelihood of memory leaks and system crashes.
For a deeper dive into Java’s core concepts, Upskillist’s online Coding & Technology course offers a comprehensive guide.
- What is the Java ClassLoader?
Java ClassLoader is part of the Java Runtime Environment that dynamically loads Java classes into the JVM. It is primarily responsible for loading classes and interfaces. There are three built-in ClassLoaders in Java:
- Bootstrap ClassLoader: This is the first classloader which is the superclass of the Extension classloader. It loads the rt.jar file, which contains all class files of Java Standard Edition like java.lang package classes, java.net package classes, java.util package classes, java.io package classes, java.sql package classes, etc.
- Extension ClassLoader: This is the child classloader of Bootstrap and parent classloader of System classloader. It loads the jar files located inside the $JAVA_HOME/jre/lib/ext directory.
- System/Application ClassLoader: This is the child classloader of Extension classloader. It loads the class files from the classpath. By default, the classpath is set to the current directory. You can change the classpath using “-cp” or “-classpath” switch. It is also known as Application classloader.
- What is the difference between an abstract class and an interface?
An abstract class and an interface are core components of Java, but they have distinct differences.
- Abstract Class: An abstract class can have both abstract (method without body) and non-abstract methods (method with body). It can have variables, constructors and static methods. An abstract class can provide the implementation of the interface. It is used to provide the default implementation of the interface methods.
- Interface: Interface only contains abstract methods, but from Java 8, it can contain default and static methods. It can have variables which are implicitly final and static. Interface supports multiple inheritance and doesn’t have constructors. It provides total abstraction; that is, all the methods in an interface are public and abstract by default.
- Explain the role of JVM in Java?
The Java Virtual Machine (JVM) plays a critical role in Java’s platform-independent nature. JVM is a part of Java Runtime Environment (JRE) and creates a runtime environment for the Java bytecode to be executed. It is responsible for converting the bytecode into machine-specific code. JVMs are platform-specific runtime implementations. Its specifications have two components: one is a regular JVM run time environment, and the other is JVM class file format, which specifies the files containing the compiled code to be used by the runtime environment.
- What are the pillars of Object-Oriented Programming (OOP) in Java?
The four pillars of OOP in Java are:
- Encapsulation: This refers to the bundling of data, along with the methods that operate on that data, into a single unit.
- Inheritance: This mechanism allows one class to inherit properties (fields and methods) from another class.
- Polymorphism: It allows one interface to be used for a general class of actions.
- Abstraction: It is a process of hiding the implementation details from the user and only providing the functionality.
- Explain the difference between JDK, JRE, and JVM?
- JDK (Java Development Kit): It is a software development environment used for developing Java applications and applets. It physically exists and contains JRE + development tools.
- JRE (Java Runtime Environment): It provides the runtime environment in which Java bytecode can be executed. It physically exists. It contains a set of libraries + other files that JVM uses at runtime.
- JVM (Java Virtual Machine): It is an abstract machine. It provides a runtime environment in which Java bytecode can be executed. It doesn’t physically exist.
The remaining questions will continue to delve into more advanced concepts, which we cover in our Upskillist online Coding & Technology course.
From understanding Java’s garbage collection and exception handling to deep-diving into Java libraries, Java I/O classes, multithreading, generics, and more, our online course offers extensive, hands-on experience to make you interview-ready.
- What is garbage collection in Java?
Garbage Collection in Java is a process that helps in memory management. It automatically reclaims the runtime unused memory. It frees up the memory by destroying the objects that are not in use.
- Explain the concept of Exception Handling in Java?
Exception handling is a powerful mechanism to handle runtime errors so that the normal flow of the application can be maintained. The core advantage of exception handling is to maintain the normal flow of the application. Exception handling in Java is performed via five keywords: try, catch, throw, throws, and finally.
- What are Java libraries?
Java libraries consist of a set of classes and interfaces that are bundled together. They provide a standard way to handle tasks like creating graphical user interfaces, reading and writing from a disk, or communicating over a network. The Java Class Library (JCL) is a standard library, which is bundled with Java. Other libraries, like Apache Commons, Google Guava, and JHipster, are also available.
- Explain Java I/O Classes.
Java I/O (Input and Output) is used to process the input and produce the output based on the input. Java uses the concept of streams to make I/O operations fast. The java.io package contains all the classes required for input and output operations.
- What is Multithreading in Java?
Multithreading is a feature that allows concurrent execution of two or more parts of a program for maximum utilisation of CPU. Threads are independent, they don’t affect the execution of other threads. An exception thrown in one thread doesn’t affect other threads.
- Explain the concept of Generics in Java.
Generics in Java is similar to templates in C++. The idea is to allow type (Integer, String, … etc., and user-defined types) to be a parameter to methods, classes, and interfaces. Using Generics, it is possible to create classes that work with different data types.
Remember, understanding the basics of Java and mastering the language are two different things. While these questions will give you a good starting point, mastering Java requires a deep understanding of these concepts, along with hands-on experience.
That’s where the Upskillist online Coding & Technology course can play a pivotal role. Designed to help aspirants like you master the intricacies of Java and other essential coding skills, this course can be your ticket to nail your next Java interview.
Whether you’re a beginner trying to build a strong foundation or a professional aiming to level up your Java skills, this course offers a flexible, immersive, and in-depth learning experience. So why wait? Enrol today and step into the world of coding and technology with confidence.
- What are Java Collections?
Java Collections Framework provides a well-designed set of classes and interfaces that represent a group of objects as a single unit. These classes and interfaces implement fundamental data structures, which are highly useful in performing more complex operations on a set of objects.
- What are anonymous inner classes?
An anonymous inner class is a local inner class without a name. It is declared and instantiated in a single statement. Anonymous inner classes are often used in graphical and multithreaded programming.
- Explain the difference between equals() method and “==” operator?
In Java, the equals() method compares the values of two objects for equality, while the “==” operator compares the references, not the values. It checks to see if two object references point to the same object in memory.
- How can we achieve thread safety in Java?
Thread safety in Java is achieved using synchronization, which ensures that only one thread can access the resource at a given point in time. This prevents data inconsistency issues from arising.
- What is JDBC?
JDBC (Java Database Connectivity) is a standard Java API for independent connectivity between the Java programming language and a wide range of databases. The JDBC API provides a call-level API for SQL-based database access.
- Explain the concept of a Java Bean?
A Java Bean is a software component that has been designed to be reusable in a variety of different environments. It is a Java class that should follow certain conventions: the class should be public, it should have a no-arg constructor, it should allow access to variables using getter and setter methods.
In your quest to land your dream job, nailing these Java programming questions can set you apart from other candidates. But, to answer these questions confidently, you need robust understanding and practice. The Upskillist online Coding & Technology course is the perfect place for you to get that.
But wait, there’s more. Let’s continue in the next section where we delve into more advanced Java programming concepts and related interview questions. Remember, the more you learn, the closer you are to acing that interview!
- What is a constructor in Java?
A constructor in Java is a special method used to initialise objects. It’s called when an instance of an object is created and its name is the same as the class name.
- What are exception handling keywords in Java?
There are four keywords used in Java for exception handling: try, catch, finally, and throw.
- What is the role of the ‘finally’ block in exception handling?
‘Finally’ block always executes, whether an exception occurs in try block or not. It is generally used to clean up resources.
- Explain the difference between an abstract class and an interface in Java?
An abstract class can have both abstract and non-abstract methods, whereas an interface can only have abstract methods. Abstract classes can have constructors, but interfaces cannot.
- Explain the concept of polymorphism in Java?
Polymorphism in Java is the ability where an object can take on many forms. It supports overriding and interface inheritance, allowing for more flexible and dynamic code.
- What is Java Serialization?
Serialisation is a mechanism to convert the state of an object into a byte stream. It is mainly used in networking programming for objects to be sent over the network.
- How does the ‘this’ keyword work in Java?
‘This’ keyword in Java is a reference variable that refers to the current object. It can be used to refer to instance variable of current class, invoke current class constructor, and to pass an argument in the method call.
- What is the difference between final, finally, and finalise in Java?
‘final’ is a keyword that is used to apply restrictions on class, method, and variable. ‘finally’ is used in exception handling and it contains all the crucial statements that must be executed whether an exception occurs or not. ‘finalise’ is a method that is invoked by the Garbage Collector before an object is being garbage collected.
- What is method overloading in Java?
Method overloading is a feature that allows a class to have two or more methods having the same name but different parameter lists. It increases the readability of the program.
These top Java interview questions cover a range of concepts and help you demonstrate a well-rounded understanding of Java programming. Practice these questions and enroll in our Online Coding & Technology Course at Upskillist to take your understanding of Java to the next level. In the next section, we’ll delve into more intricate concepts and questions. Stay tuned!
- Explain the concept of method overriding in Java?
Method overriding allows a subclass to provide a specific implementation of a method that is already provided by its superclass. This is used for runtime polymorphism and the method must have the same name, return type, and parameters.
- What is an anonymous class in Java?
An anonymous class is a class defined without a name in a single line of code using new keyword. It’s useful when you need to use a local class only once while creating an object.
- What is a Java enum?
Enums are a type of class in Java that have a fixed set of constants. They are used when we know all possible values at compile time, such as the days of the week, states of a game, etc. Enums increase compile-time safety and reduce the possibility of errors.
- How can you create a thread in Java?
You can create a thread in Java by either extending the Thread class or implementing the Runnable interface. Then you need to override the run() method and call start() method to begin execution of the thread.
- What is synchronization in Java?
Synchronisation in Java is the capability to control the access of multiple threads to any shared resource. It is used to prevent thread interference and consistency problem.
- What is the difference between ArrayList and LinkedList in Java?
Both ArrayList and LinkedList are implementations of the List interface. ArrayList uses a dynamic array for storage, offering fast random access and efficient operations when resizing is infrequent. LinkedList, on the other hand, is implemented as a double-linked list. Its performance is better in adding or removing from the beginning or middle of the list.
- What is a Java package and how is it used?
A package in Java is a namespace that organises a set of related classes and interfaces. It helps to keep the class name space compartmentalized. It can be imported in other classes using the import keyword.
- Explain the Java API and how it’s used?
Java API is a library of pre-written classes, which are free to use. It’s included in the JDK. It helps developers to handle common programming tasks such as string handling, network communication, etc.
- What is the JDBC API and how is it used?
Java Database Connectivity (JDBC) is an API that lets you access virtually any tabular data source from a Java application. It’s used to connect to a database, execute SQL statements and retrieve results.
- How does the Java Virtual Machine (JVM) work?
The Java Virtual Machine (JVM) is a virtual machine that enables a computer to run Java programs. JVM is responsible for loading, verifying, and executing the byte code, as well as managing resources.
These were some of the top Java interview questions that will help you prepare for your interviews. As you can see, Java is an extensive language with a multitude of features. If you feel the need for more practice or wish to learn more about Java, consider enrolling in our Online Coding & Technology Course at Upskillist. It will enhance your knowledge and give you hands-on experience to ace your Java interview.
In the next section, we will dive into more advanced Java concepts. Stay tuned!
- What are the differences between == and equals() in Java?
In Java, == is used to compare the reference of two variables while equals() method is used for comparing the values of two objects.
- What is an exception in Java?
An exception in Java represents a problem that occurs during a program’s execution. It is an event that disrupts the normal flow of the program’s instructions.
- What is the difference between checked and unchecked exceptions in Java?
Checked exceptions are checked at compile-time. It means if a method is throwing a checked exception, it should handle the exception using a try-catch block or it should declare the exception using the throws keyword. On the other hand, unchecked exceptions are not checked at compile-time, but they are checked at runtime.
- What is the purpose of finally block in Java?
In Java, finally block is used to put important code, it will be executed whether an exception is handled or not. So, it’s mainly used to put clean up code.
- What is serialization in Java?
Serialization in Java is a mechanism of writing the state of an object into a byte stream. It’s mainly used in Hibernate, RMI, JPA, EJB and JMS technologies.
- How can we run a JAR file in Java?
A JAR file in Java can be run using the command prompt by using the command: java -jar filename.jar.
- What are Wrapper Classes in Java?
In Java, wrapper classes are the Object representation of eight primitive types. They convert primitive data types into objects. Objects are needed if we wish to modify the arguments passed into a method.
- What is Autoboxing and Unboxing in Java?
Autoboxing is the automatic conversion of primitive types to the object of their corresponding wrapper classes. Unboxing is the conversion of an object of a wrapper type to its corresponding primitive value.
- What is the purpose of default constructor in Java?
In Java, a default constructor is a no-arg constructor automatically provided by the compiler if no other constructors are provided. It initialises the instance variables with default values.
- What is Singleton class in Java?
Singleton class is a class whose only one instance can be created at any given time, in one JVM. A class can be made Singleton by making its constructor private.
These top Java interview questions should give you a good foundation for your Java interviews. As you can see, Java is a diverse and complex language. If you want to further expand your knowledge and become proficient in Java, consider enrolling in our Online Coding & Technology Course at Upskillist. This comprehensive course will provide you with hands-on experience and in-depth understanding of Java.
- What is the Java Reflection API? Why is it so important?
Java’s Reflection API allows a program to inspect and manipulate itself or another program (code) internally. This feature is useful for developing debugging tools, testing frameworks, advanced libraries or simply interacting with unfamiliar code.
- What is the diamond operator in Java?
The diamond operator (<>) was introduced in Java 7 to simplify the use of generics when creating an object. It allows the compiler to infer the type parameters from the context, reducing verbosity and making the code easier to read.
- How can we convert a string to an integer in Java?
In Java, we can convert a string to an integer using the Integer.parseInt() or Integer.valueOf() method. For example, Integer.parseInt(“123”) would return the integer 123.
- How can we convert an integer to a string in Java?
In Java, we can convert an integer to a string using the Integer.toString() method or String.valueOf() method. For example, Integer.toString(123) would return the string “123”.
- What is Java’s ternary operator?
The ternary operator is a shorthand way of doing if-else statements in Java. It’s a one-liner replacement for if-then-else statement and used a lot in java programming. The syntax is: variable num = (expression) ? value if true : value if false.
- What are Java Annotations?
Java Annotations are a form of metadata that provide data about a program that is not part of the program itself. They have no direct effect on the operation of the code they annotate.
- Can you explain the concept of Java Generics?
Generics in Java is a language feature that allows the use of type parameters when defining classes, interfaces, and methods. By using generics, programmers can implement generic algorithms that work on collections of different types, can be checked at compile time, and do not require casts or comments to explain their behaviour.
- What is the Java Stream API?
The Java Stream API was added in Java 8. It is used to process collections of objects. A stream is a sequence of objects that supports various methods that can be pipelined to produce the desired result.
- What are the benefits of using the Java Collection Framework?
The Java Collection Framework provides a well-designed set of classes and interfaces for storing and manipulating a group of data as a single unit. It provides many benefits such as reducing programming effort, increasing performance, providing interoperability between unrelated APIs, reducing the effort to learn and to use new APIs, and fostering software reuse.
- What are Java Lambdas and Functional Interfaces?
Introduced in Java 8, lambda expressions are a new language feature which allows us to treat functionality as a method argument or code as data. A functional interface is an interface that contains only one abstract method and is used as the basis for lambda expressions in functional programming.
Conclusion
By understanding these top Java interview questions, you’ll be better prepared for your technical interview. Java’s wide applicability and versatility make it a desirable skill in the tech industry. Upskillist’s Online Coding & Technology Course is designed to help you master Java and increase your chances of acing your interview. This course is filled with rich content and practical exercises that are essential for every aspiring Java developer. It covers all the basic concepts of Java for the interview and beyond, to ensure you are well-equipped for your Java programming journey.
Remember, practice is key when it comes to programming. Keep coding, keep exploring, and good luck with your Java interviews!